If you also want to design the login and signup page in Android Studio and you are not able to give it a material design, then you can create this design and put it in your application. This application design is very beautiful and very clean design which is very easy to make.
It is very easy, I am giving its code to all of you below, which you can copy and use in your Android Studio project and create a beautiful login and sign-up page for your application.
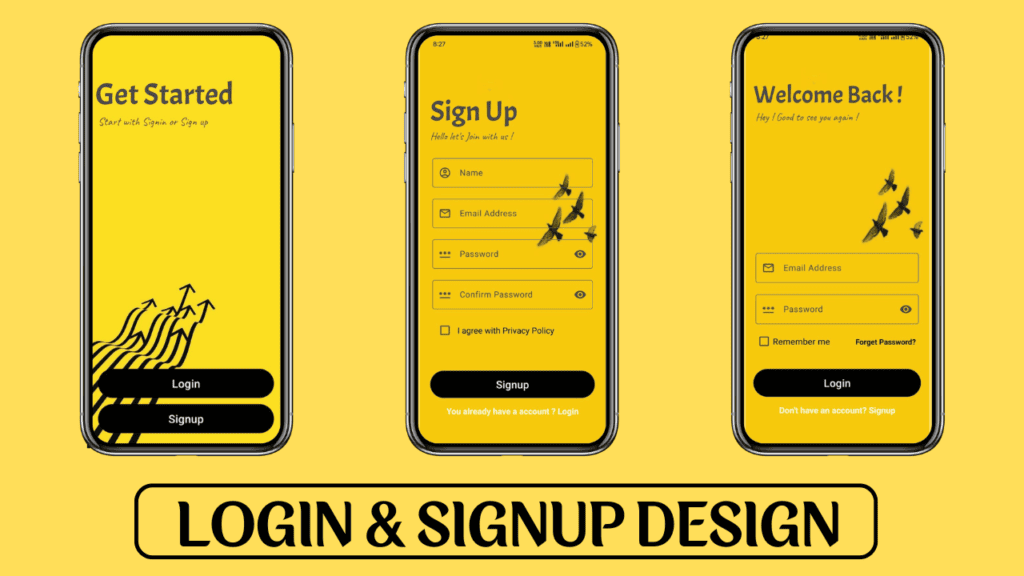
How to Create a Login and Signup Page in Android Studio
Creating a login and signup screen design in Android Studio based on the provided design image involves several steps. Below is a detailed guide on how to achieve this, along with the necessary XML and Java code snippets.
Background Image
We are using some images for this project, we are giving that image below, you can download it and use it in your project or you can use your own created image in place of that image. !
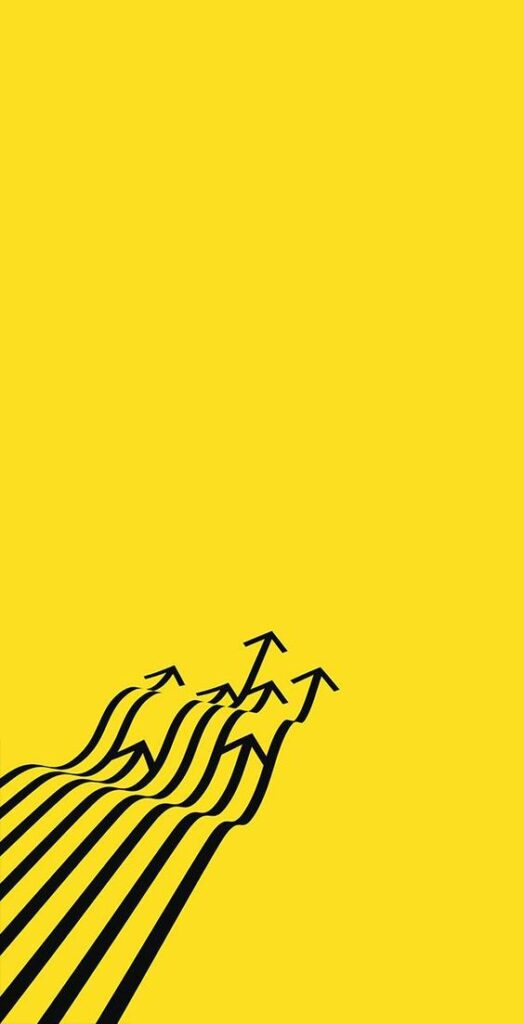
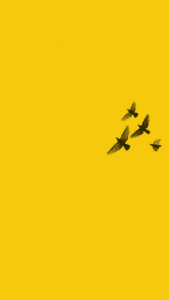
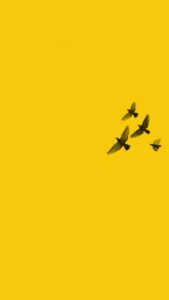
You have to download all these images and put them inside the drawable folder of your project.
Step 1: Setting Up the Project
- Create a New Project: Open Android Studio and create a new project with an Empty Activity. If you already have a project, then open it in your Android Studio.
- Configure the Project: Name your project (e.g., “LoginSignupDesign”), choose the language (Java/Kotlin), and set the minimum SDK.
Step 2: Add Dependencies
First of all, you have to add the dependencies of Material Design in your project’s ” gradle.build”.
implementation libs.material.v150
Step 3: Designing the Layouts
We will create three XML layout files for the different screens: activity_main.xml
, activity_login.xml
, and activity_signup.xml
.
Read More:- How to add Lottie Animation in the Android App and Make Professional
1. activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/main"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@drawable/background"
tools:context=".MainActivity">
<!-- Text above the button -->
<TextView
android:padding="15dp"
android:id="@+id/textView"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Get Started"
android:textSize="54sp"
android:fontFamily="@font/acme"
android:layout_centerHorizontal="true"
android:layout_marginTop="100dp"/>
<TextView
android:padding="15dp"
android:id="@+id/textView2"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text=" Start with Signin or Sign up"
android:textSize="20sp"
android:fontFamily="@font/caveat"
android:layout_centerHorizontal="true"
android:layout_marginTop="170dp"/>
<!-- Button at the bottom -->
<com.google.android.material.button.MaterialButton
android:id="@+id/loginButton"
android:layout_width="match_parent"
android:layout_height="60dp"
android:text="Login"
android:textSize="20dp"
android:layout_alignParentBottom="true"
android:layout_marginStart="20dp"
android:layout_marginEnd="20dp"
android:layout_marginBottom="95dp"
android:backgroundTint="#000000"/>
<com.google.android.material.button.MaterialButton
android:id="@+id/signButton"
android:layout_width="match_parent"
android:layout_height="60dp"
android:textSize="20dp"
android:text="Signup"
android:layout_alignParentBottom="true"
android:layout_marginStart="20dp"
android:layout_marginEnd="20dp"
android:layout_marginBottom="32dp"
android:backgroundTint="@color/black"/>
</RelativeLayout>
MainActivity.java
import android.content.Intent;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import androidx.activity.EdgeToEdge;
import androidx.appcompat.app.AppCompatActivity;
import androidx.core.graphics.Insets;
import androidx.core.view.ViewCompat;
import androidx.core.view.WindowInsetsCompat;
public class MainActivity extends AppCompatActivity {
Button signup,login;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
EdgeToEdge.enable(this);
setContentView(R.layout.activity_main);
signup=findViewById(R.id.signButton);
login=findViewById(R.id.loginButton);
signup.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Intent intent= new Intent(MainActivity.this,Login.class);
startActivity(intent);
}
});
login.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Intent intent= new Intent(MainActivity.this,Signup.class);
startActivity(intent);
}
});
}
}
2. activity_login.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/main"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:background="@drawable/loginback"
tools:context=".Login">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="bottom"
android:layout_marginBottom="60dp"
android:orientation="vertical">
<TextView
android:id="@+id/textView"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Sign Up"
android:layout_marginStart="20dp"
android:textSize="54sp"
android:fontFamily="@font/acme"
android:layout_centerHorizontal="true"/>
<TextView
android:layout_marginStart="20dp"
android:id="@+id/textView2"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Hello let's Join with us !"
android:textSize="20sp"
android:fontFamily="@font/caveat"
android:layout_centerHorizontal="true" />
<com.google.android.material.textfield.TextInputLayout
android:id="@+id/nameLayout"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginStart="24dp"
app:startIconDrawable="@drawable/account_circle24px"
android:layout_marginTop="24dp"
android:layout_marginEnd="24dp"
android:hint="Name">
<com.google.android.material.textfield.TextInputEditText
android:id="@+id/nameEditText"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:inputType="textPersonName"/>
</com.google.android.material.textfield.TextInputLayout>
<com.google.android.material.textfield.TextInputLayout
android:id="@+id/emailLayout"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginStart="24dp"
android:layout_marginTop="16dp"
app:startIconDrawable="@drawable/mail_24px"
android:layout_marginEnd="24dp"
android:hint="Email Address">
<com.google.android.material.textfield.TextInputEditText
android:id="@+id/emailEditText"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:inputType="textEmailAddress"/>
</com.google.android.material.textfield.TextInputLayout>
<com.google.android.material.textfield.TextInputLayout
android:id="@+id/passwordLayout"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginStart="24dp"
android:layout_marginTop="16dp"
app:startIconDrawable="@drawable/password_24px"
app:endIconMode="password_toggle"
android:layout_marginEnd="24dp"
android:hint="Password">
<com.google.android.material.textfield.TextInputEditText
android:id="@+id/passwordEditText"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:inputType="textPassword"/>
</com.google.android.material.textfield.TextInputLayout>
<com.google.android.material.textfield.TextInputLayout
android:id="@+id/confirmPasswordLayout"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginStart="24dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="24dp"
app:startIconDrawable="@drawable/password_24px"
app:endIconMode="password_toggle"
android:hint="Confirm Password">
<com.google.android.material.textfield.TextInputEditText
android:id="@+id/confirmPasswordEditText"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginBottom="10dp"
android:inputType="textPassword"/>
</com.google.android.material.textfield.TextInputLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal"
android:gravity="center_vertical"
android:layout_marginTop="6dp"
android:layout_marginStart="24dp"
android:layout_marginBottom="25dp"
android:layout_marginEnd="24dp">
<CheckBox
android:id="@+id/agreeCheckBox"
android:layout_width="wrap_content"
android:layout_height="wrap_content"/>
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="I agree with Privacy Policy"
android:textSize="16sp"
android:textColor="@android:color/black"/>
</LinearLayout>
<com.google.android.material.button.MaterialButton
android:id="@+id/signButton"
android:layout_width="match_parent"
android:layout_height="60dp"
android:layout_marginStart="20dp"
android:layout_marginEnd="20dp"
android:layout_marginTop="24dp"
android:text="Signup"
android:textSize="20sp"
android:textAllCaps="false"
android:backgroundTint="@color/black"/>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal"
android:gravity="center_vertical"
android:layout_gravity="center"
android:layout_marginTop="10dp">
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="You already have a account ? Login"
android:textSize="16sp"
android:textAlignment="center"
android:textStyle="bold"
android:textColor="@color/white"/>
</LinearLayout>
</LinearLayout>
</LinearLayout>
3. activity_signup.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/main"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:background="@drawable/loginback"
tools:context=".Signup">
<LinearLayout
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="70dp"
android:orientation="vertical">
<TextView
android:id="@+id/textView"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Welcome Back !"
android:layout_marginStart="20dp"
android:textSize="45dp"
android:fontFamily="@font/acme"
android:layout_centerHorizontal="true"/>
<TextView
android:id="@+id/textView2"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Hey ! Good to see you again !"
android:layout_marginStart="24dp"
android:textSize="20sp"
android:fontFamily="@font/caveat"
android:layout_centerHorizontal="true" />
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_marginBottom="60dp"
android:gravity="bottom"
android:orientation="vertical">
<com.google.android.material.textfield.TextInputLayout
android:id="@+id/emailLayout"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginStart="24dp"
android:layout_marginTop="16dp"
app:startIconDrawable="@drawable/mail_24px"
android:layout_marginEnd="24dp"
android:hint="Email Address">
<com.google.android.material.textfield.TextInputEditText
android:id="@+id/emailEditText"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:inputType="textEmailAddress"/>
</com.google.android.material.textfield.TextInputLayout>
<com.google.android.material.textfield.TextInputLayout
android:id="@+id/passwordLayout"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginStart="24dp"
android:layout_marginTop="16dp"
app:startIconDrawable="@drawable/password_24px"
app:endIconMode="password_toggle"
android:layout_marginEnd="24dp"
android:hint="Password">
<com.google.android.material.textfield.TextInputEditText
android:id="@+id/passwordEditText"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:inputType="textPassword"/>
</com.google.android.material.textfield.TextInputLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal">
<CheckBox
android:id="@+id/rememberPasswordCheckBox"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Remember me"
android:textSize="16sp"
android:textColor="@color/black"
android:layout_marginStart="24dp"
android:layout_marginTop="8dp"
android:layout_marginEnd="24dp"
android:checked="false"/>
<!-- Forget Password Text -->
<TextView
android:id="@+id/forgetPasswordText"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Forget Password?"
android:textSize="14sp"
android:textStyle="bold"
android:textColor="@color/black"
android:layout_marginStart="24dp"
android:layout_marginTop="8dp"
android:layout_marginEnd="24dp"/>
</LinearLayout>
<!-- Remember Password CheckBox -->
<com.google.android.material.button.MaterialButton
android:id="@+id/signButton"
android:layout_width="match_parent"
android:layout_height="60dp"
android:layout_marginStart="20dp"
android:layout_marginEnd="20dp"
android:layout_marginTop="24dp"
android:text="Login"
android:textSize="20sp"
android:textAllCaps="false"
android:backgroundTint="@color/black"/>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal"
android:gravity="center_vertical"
android:layout_gravity="center"
android:layout_marginTop="10dp">
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Don't have an account? Signup"
android:textSize="16sp"
android:textAlignment="center"
android:textStyle="bold"
android:textColor="@color/white"/>
</LinearLayout>
</LinearLayout>
</LinearLayout>
This was the design of our Login and Signup Page, by copying which you can easily design the Login and Signup Page of your project. And it is a very beautiful design which gives a professional look to your project!