Hello friends, today I am going to tell you all how you can install the Intro Slider inside your Android application. It is very easy to install!
The Intro Slider is placed at the beginning of the application and we get to see it when we start the application for the first time and it consists of one-two-three or four pages on which we are told some information about the application and is quite beautiful to look at. What happens is that as you slide, you get to see all the information and as you reach the last page, you reach the Mainactivity.
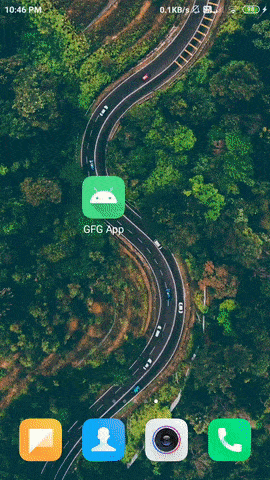
What we are going to Create in this article?
Today, after the splash screen inside an application, we will show an intro slider that will appear when the application starts for the first time and it will have three pages that after completion will go directly to the home activity! And when the application is restarted, it will appear directly as HomeActivity after the splash screen!
Step-by-Step Implementation of Intro Slider in Android App?
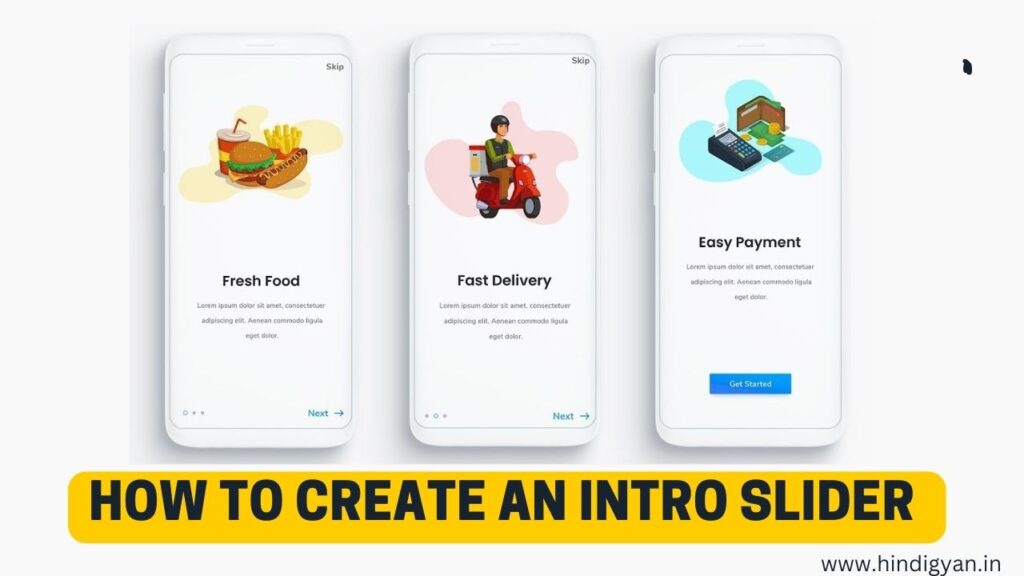
Step 1: Create a New Project
First of all, we have to create a new project using Java language inside Android Studio or if you already have a project then you have to open it inside Android Studio.
Step 2: Adding the dependency for Picasso in your build.gradle file
In the intro slider that we are going to create, we are going to show the image for which we have to add the dependency of Picasso inside our project which is given below and we have to paste it inside the build.gradle of the project. This image is going to be visible in our project via online URL
implementation 'com.squareup.picasso:picasso:2.71828'
Step 3: Adding permissions for the Internet
We are going to display the photos via an online URL in this Intro Slider Project! For which we will need internet, for this we need to give internet permission in the Androidmanifest.xml file of the project. For that, you have to open the Androidmanifest.xml file and give internet permission.
<uses-permission android:name="android.permission.INTERNET"/>
Step 4: Create Empty Activity for IntroSlider
To create an intro slider, you have to create a separate intro slider activity in your project, for which we will need an empty activity, you can create a new empty activity by going to app > Right Click > New > Activity > Empty View Activity.
Step 4.1: Working with the intro_slider.xml file
Navigate to the app > res > layout > intro_slider.xml and add the below code to that file. Below is the code for the intro_slider.xml file.
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:weightSum="5"
tools:context=".MainActivity">
<!--view pager for displaying our slides-->
<androidx.viewpager.widget.ViewPager
android:id="@+id/idViewPager"
android:layout_width="match_parent"
android:layout_height="match_parent" />
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_alignParentBottom="true"
android:background="@android:color/transparent"
android:orientation="horizontal"
android:weightSum="5">
<View
android:layout_width="0dp"
android:layout_height="0dp"
android:layout_weight="2" />
<!--adding linear layout for
creating dots view-->
<LinearLayout
android:id="@+id/idLLDots"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_gravity="center_horizontal"
android:layout_weight="1"
android:gravity="center_horizontal"
android:orientation="horizontal" />
<!--button for skipping our intro slider-->
<Button
android:id="@+id/idBtnSkip"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_margin="10dp"
android:layout_weight="2"
android:background="@color/purple_500"
android:backgroundTint="@color/purple_500"
android:text="Skip"
android:textAllCaps="false"
android:textColor="@color/white" />
</LinearLayout>
</RelativeLayout>
Step 5: Create a layout file for our slider item
Now we will create an item that we will be displaying in our slider. So for creating a new layout navigate to the app > res > layout > Right-click on it > Click on New > layout resource file and name it as slider_layout.xml and add below code to it.
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/idRLSlider"
android:layout_width="match_parent"
android:layout_height="match_parent">
<!--text view for displaying
our heading-->
<TextView
android:id="@+id/idTVtitle"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_margin="20dp"
android:padding="10dp"
android:text="Slide 1"
android:textAlignment="center"
android:textColor="@color/white"
android:textSize="20sp"
android:textStyle="bold" />
<!--Image view for displaying
our slider image-->
<ImageView
android:id="@+id/idIV"
android:layout_width="200dp"
android:layout_height="200dp"
android:layout_below="@id/idTVtitle"
android:layout_centerHorizontal="true"
android:layout_marginTop="50dp"
android:padding="10dp"
android:src="@mipmap/ic_launcher" />
<!--text view for displaying our
slider description-->
<TextView
android:id="@+id/idTVheading"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_below="@id/idIV"
android:layout_marginStart="20dp"
android:layout_marginTop="90dp"
android:layout_marginEnd="20dp"
android:padding="10dp"
android:text="C++ data structure and ALgorithm Course"
android:textAlignment="center"
android:textColor="@color/white"
android:textSize="15sp" />
</RelativeLayout>
Step 6: Creating a Modal class for storing all the data for Slider items
For creating a new Modal class navigate to the app > java > your app’s package name > Right-click on it and click on New > Java class and name it as SliderModal.java After creating this class add the below code to it.
public class SliderModal {
// string variable for storing
// title, image url and description.
private String title;
private String heading;
private String imgUrl;
private int backgroundDrawable;
public SliderModal() {
// empty constructor is required
// when using firebase
}
// creating getter methods.
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public String getHeading() {
return heading;
}
// creating setter methods
public void setHeading(String heading) {
this.heading = heading;
}
public String getImgUrl() {
return imgUrl;
}
public void setImgUrl(String imgUrl) {
this.imgUrl = imgUrl;
}
// constructor for our modal class
public SliderModal(String title, String heading, String imgUrl, int backgroundDrawable) {
this.title = title;
this.heading = heading;
this.imgUrl = imgUrl;
this.backgroundDrawable = backgroundDrawable;
}
public int getBackgroundDrawable() {
return backgroundDrawable;
}
public void setBackgroundDrawable(int backgroundDrawable) {
this.backgroundDrawable = backgroundDrawable;
}
}
Step 7: Create an Adapter class for setting data to each view
For creating a new Adapter class navigate to the app > java > your app’s package name > Right-click on it > New Java class and name it as SliderAdapter and add the below code to it. Comments are added inside the code to understand the code in more detail.
import android.content.Context;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.ImageView;
import android.widget.RelativeLayout;
import android.widget.TextView;
import androidx.annotation.NonNull;
import androidx.viewpager.widget.PagerAdapter;
import com.squareup.picasso.Picasso;
import java.util.ArrayList;
public class SliderAdapter extends PagerAdapter {
// creating variables for layout
// inflater, context and array list.
LayoutInflater layoutInflater;
Context context;
ArrayList<SliderModal> sliderModalArrayList;
// creating constructor.
public SliderAdapter(Context context, ArrayList<SliderModal> sliderModalArrayList) {
this.context = context;
this.sliderModalArrayList = sliderModalArrayList;
}
@Override
public int getCount() {
// inside get count method returning
// the size of our array list.
return sliderModalArrayList.size();
}
@Override
public boolean isViewFromObject(@NonNull View view, @NonNull Object object) {
// inside isViewFromobject method we are
// returning our Relative layout object.
return view == (RelativeLayout) object;
}
@NonNull
@Override
public Object instantiateItem(@NonNull ViewGroup container, int position) {
// in this method we will initialize all our layout
// items and inflate our layout file as well.
layoutInflater = (LayoutInflater) context.getSystemService(context.LAYOUT_INFLATER_SERVICE);
// below line is use to inflate the
// layout file which we created.
View view = layoutInflater.inflate(R.layout.slider_layout, container, false);
// initializing our views.
ImageView imageView = view.findViewById(R.id.idIV);
TextView titleTV = view.findViewById(R.id.idTVtitle);
TextView headingTV = view.findViewById(R.id.idTVheading);
RelativeLayout sliderRL = view.findViewById(R.id.idRLSlider);
// setting data to our views.
SliderModal modal = sliderModalArrayList.get(position);
titleTV.setText(modal.getTitle());
headingTV.setText(modal.getHeading());
Picasso.get().load(modal.getImgUrl()).into(imageView);
// below line is to set background
// drawable to our each item
sliderRL.setBackground(context.getResources().getDrawable(modal.getBackgroundDrawable()));
// after setting the data to our views we
// are adding the view to our container.
container.addView(view);
// at last we are
// returning the view.
return view;
}
@Override
public void destroyItem(@NonNull ViewGroup container, int position, @NonNull Object object) {
// this is a destroy view method
// which is use to remove a view.
container.removeView((RelativeLayout) object);
}
}
Step 8: Creating a custom gradient drawable for the background of each slide
Navigate to the app > res > drawable > Right-click on it > New > drawable resource file and name the file as gradient_one and add the below code to it.
gradient_one.xml
<?xml version="1.0" encoding="utf-8"?>
<shape xmlns:android="http://schemas.android.com/apk/res/android"
android:shape="rectangle">
<gradient
android:angle="90"
android:endColor="@color/purple_500"
android:startColor="#4B6CD6" />
</shape>
gradient_two.xml
<?xml version="1.0" encoding="utf-8"?>
<shape xmlns:android="http://schemas.android.com/apk/res/android"
android:shape="rectangle">
<gradient
android:angle="90"
android:endColor="#FF9800"
android:startColor="#F4C22B" />
</shape>
gradient_three.xml
<?xml version="1.0" encoding="utf-8"?>
<shape xmlns:android="http://schemas.android.com/apk/res/android"
android:shape="rectangle">
<gradient
android:angle="90"
android:endColor="#F44336"
android:startColor="#FD7B52" />
</shape>
Step 9: Working with the IntroSlider.java file
Go to the IntroSlider.java file and refer to the following code. Below is the code for the IntroSlider.java file. Comments are added inside the code to understand the code in more detail.
import androidx.appcompat.app.AppCompatActivity;
import androidx.viewpager.widget.ViewPager;
import android.content.Intent;
import android.content.SharedPreferences;
import android.os.Bundle;
import android.os.Handler;
import android.view.View;
import android.widget.Button;
import android.widget.LinearLayout;
import android.widget.TextView;
import java.util.ArrayList;
public class IntroSlider extends AppCompatActivity {
SliderAdapter adapter;
Button skip;
int size;
private ViewPager viewPager;
private LinearLayout dotsLL;
private ArrayList<SliderModal> sliderModalArrayList;
private TextView[] dots;
// creating a method for view pager for on page change listener.
ViewPager.OnPageChangeListener viewListener = new ViewPager.OnPageChangeListener() {
@Override
public void onPageScrolled(int position, float positionOffset, int positionOffsetPixels) {
}
@Override
public void onPageSelected(int position) {
// we are calling our dots method to
// change the position of selected dots.
addDots(size, position);
if (position == 2) {
// Navigate to another activity here.
new Handler().postDelayed(new Runnable() {
@Override
public void run() {
Intent intent = new Intent(IntroSlider.this, MainActivity.class);
intent.putExtra("linkss","https://hssakingamapp.blogspot.com/");
startActivity(intent);
// Optionally, you can finish the current activity to prevent going back.
finish();
}
},1000);
}
}
@Override
public void onPageScrollStateChanged(int state) {
}
};
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_intro_slider);
SharedPreferences preferences = getSharedPreferences("PREFERENCE",MODE_PRIVATE);
String FristTime = preferences.getString("FirstInstall","");
if (FristTime.equals("Yes")){
Intent intent = new Intent(IntroSlider.this,MainActivity.class);
intent.putExtra("linkss","https://hssakingamapp.blogspot.com/");
startActivity(intent);
}else {
SharedPreferences.Editor editor = preferences.edit();
editor.putString("FirstInstall", "Yes");
editor.apply();
}
skip=findViewById(R.id.idBtnSkip);
skip.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Intent intent=new Intent(IntroSlider.this,MainActivity.class);
intent.putExtra("linkss","https://hssakingamapp.blogspot.com/");
startActivity(intent);
finish();
}
});
// initializing all our views.
viewPager = findViewById(R.id.idViewPager);
dotsLL = findViewById(R.id.idLLDots);
// in below line we are creating a new array list.
sliderModalArrayList = new ArrayList<>();
// on below 3 lines we are adding data to our array list.
sliderModalArrayList.add(new SliderModal("Welcome", "Thank you for installing our App", "https://images.unsplash.com/photo-1610842546881-b282c580b51d?ixid=MXwxMjA3fDB8MHxlZGl0b3JpYWwtZmVlZHw5fHx8ZW58MHx8fA%3D%3D&ixlib=rb-1.2.1&auto=format&fit=crop&w=500&q=60", R.drawable.gradient_one));
sliderModalArrayList.add(new SliderModal("HSS Akingam", "The School that inspires you", "https://images.unsplash.com/photo-1610783131813-475d08664ef6?ixid=MXwxMjA3fDB8MHxlZGl0b3JpYWwtZmVlZHwxMnx8fGVufDB8fHw%3D&ixlib=rb-1.2.1&auto=format&fit=crop&w=500&q=60", R.drawable.gradient_two));
sliderModalArrayList.add(new SliderModal("App Features", "Online Registration, E-Content, Attendance Monitoring, Results, School Profile, & School Events etc", "https://images.unsplash.com/photo-1610832958506-aa56368176cf?ixid=MXwxMjA3fDB8MHxlZGl0b3JpYWwtZmVlZHwxN3x8fGVufDB8fHw%3D&ixlib=rb-1.2.1&auto=format&fit=crop&w=500&q=60", R.drawable.gradient_three));
// below line is use to add our array list to adapter class.
adapter = new SliderAdapter(IntroSlider.this, sliderModalArrayList);
// below line is use to set our
// adapter to our view pager.
viewPager.setAdapter(adapter);
// we are storing the size of our
// array list in a variable.
size = sliderModalArrayList.size();
// calling method to add dots indicator
addDots(size, 0);
// below line is use to call on
// page change listener method.
viewPager.addOnPageChangeListener(viewListener);
}
private void addDots(int size, int pos) {
// inside this method we are
// creating a new text view.
dots = new TextView[size];
// below line is use to remove all
// the views from the linear layout.
dotsLL.removeAllViews();
// running a for loop to add
// number of dots to our slider.
for (int i = 0; i < size; i++) {
// below line is use to add the
// dots and modify its color.
dots[i] = new TextView(this);
dots[i].setText(".");
dots[i].setTextSize(35);
// below line is called when the dots are not selected.
dots[i].setTextColor(getResources().getColor(R.color.black));
dotsLL.addView(dots[i]);
}
if (dots.length > 0) {
// this line is called when the dots
// inside linear layout are selected
dots[pos].setTextColor(getResources().getColor(R.color.purple_200));
}
}
}
In today’s article, we learned how you can add an intro slider in your Android Studio project. You have got all the codes here which you can copy and paste to create a good intro slider.
UPI Transaction Delay 4 Hours | Government Takes Action on UPI